Wednesday, July 29, 2009
C/SIDE Concepts
Properties
Properties control the appearance and behavior of the application objects and all their sub-objects. Properties are used to control the appearance of data, specify default values, specify colors, and define relationships.
C/AL
C/AL is the language you use to write functions in C/SIDE. In the previous table, "C/AL" refers to functions written in this language.
Triggers
A trigger is a mechanism that is built into an application object. When certain actions are performed on the application object, the trigger initiates an action. You can add your own C/AL code to the trigger to modify the default behavior of the application object or extend its functionality.
Keys
Keys define the order in which data is stored in your tables. You can speed up searches in tables by defining several keys which sort information in different ways.
Fields
A field is the smallest building block in your database. A field typically stores information such as a name or a number.
Controls
Controls are objects on a form or report that display data, perform actions, or enhance the appearance of the form, such as command buttons and text labels.
Request Form
A request form is a form that is used in a report. Before a report is run, a request form is displayed to let the user specify filters and options for the report.
Template
A template defines the overall layout of a report.
Data Items
A data item is a building block for defining a model of your data when you create a report. You use a hierarchy of data items to define which data the report will contain. A data item represents a table, and when you run a report, the system cycles through the records in the associated table. A data item can have one or more sections.
Sections
A section is a substructure of a data item, where you place controls to display information. You will typically use sections that define the body, header, and footer in your report.
Object-Oriented vs. Object-Based
Object Designer
. Design new tables, forms, pages, reports, dataports, XMLports, menusuites and codeunits.
. View existing application objects.
. Modify existing application objects.
. Run an application object (with the exception of XMLports, menusuite objects and pages).
Tools in the Object Designer
The following table lists the tools that are available in the Object Designer.
Tool - Designed for
Table Designer - Tables
Form Designer - Forms
Page Designer - Pages
Report Designer - Reports
Dataport Designer - Dataports
XMLport Designer - XMLports
C/AL editor - Codeunits
Navigation Pane Designer - MenuSuite objects
Monday, July 27, 2009
C/SIDE Fundamentals
C/SIDE applications are based on certain application objects. They are
Table
You use tables to store data. For example, a business application usually contains a customer table that stores information about each customer.Understanding tables is the key to using all the other objects.
Form
You use forms to access the information that is stored in the tables. You use forms when you enter new information and when you view information that already exists in the database.
Report
You use reports to present information. You use filters and sorting to select the data that you want to present in a report.
Dataport
You use dataports to import data from and export data to external text files.
Codeunit
A codeunit contains user-defined functions written in C/AL code. C/AL is the application language you use to write functions in C/SIDE. The functions that a codeunit contains can be used from the other objects in your application. This helps to minimize application size because the same code can be reused.
MenuSuite
A MenuSuite object contains the set of menus that are displayed in the Navigation Pane.
Page
Pages have the same functionality as forms but are intended to run on the RoleTailored client. A page can be role tailored, to suite a users needs.
Zoom Funtion In Navision
WITH Statements
and field name: [Record].[Field]
you work continuously with the same record, you can use WITH statements. When you use a WITH statement, you only have to specify the record name once.
Within the scope of [Statement], fields in [Record] can be addressed without having to specify the record name.
You can nest several WITH statements. In case of identical names, the inner WITH overrules the outer WITH-statements.
Saturday, July 25, 2009
FOR,WHILE,REPEAT
A repetitive statement is also known as a loop. The Different Loop statements are Given below.
1. FOR
2. WHILE
3. REPEAT
FOR
Repeats the inner statement until a counter variable equals the maximum or minimum value specified.
The Syntax For The above control structures are given below.
FOR [Control Variable] := [Start Number] TO [End Number] DO
[Statement]
The data type of [Control Variable], [Start Number], and [End Number] must be Boolean, number, time, or date.
Use FOR statements when you want to execute code a specific number of times.
Use a control variable to control the number of times the code is executed. The control variable can be increased or decreased by one, depending on whether TO or DOWNTO is used.
When using a FOR TO loop, the [Statement] will not be executed if the [Start Number] is greater than the end value. Likewise, when using a FOR DOWNTO loop, the [Statement] will not be executed if the [Start Number] is less than the end value.
For Eg:
1. Consider a variable 'I' for Loop.
FOR I=1 To 5 DO
Statement 1;
2. The following example shows how to nest FOR statements.
FOR I := 1 TO 5 DO
FOR J := 1 TO 7 DO
A[I,J] := 23;
Thursday, July 23, 2009
IF THEN ELSE in Navision
By using a conditional statement, you can specify a condition and one or more commands that should be executed, according to whether the condition is evaluated as TRUE or FALSE. There are two types of conditional statements in C/AL:
1. IF THEN [ELSE], where there are two choices
2. CASE, where there are more than two choices.
IF THEN ELSE statements have the following syntax.
This means that if (Condition) is true, then (Statement1) is executed. If (Condition) is false, then (Statement2) is executed.
The square brackets around ELSE
You can build even more complex control structures by nesting IF THEN ELSE statements. The following example is a typical IF THEN ELSE statement.
If 'Condition1' is false, then nothing is executed. If 'Condition1' and 'Condition2' are both true, then 'Statement1' is executed. If 'Condition1' is true and 'Condition2' is false, then 'Statement2' is executed.
The following example shows an IF statement without the optional ELSE part.
The following example shows an IF statement with the optional ELSE part.
A common error is to put an extraneous semicolon at the end of a line before an ELSE (line 4). As mentioned earlier, this is not valid according to the syntax of C/AL. The semicolon is used as a statement separator. (The end of line 4 is inside the inner IF statement.)
CASE statements have the following syntax.
1. The 'Expression' is evaluated, and the first matching value set executes the associated statement, if there is one.
2. If none of the value sets matches the value of the expression, and the optional ELSE part has been omitted, no action is taken. If the optional ELSE part is used, then the associated statement is executed.
The data type of the value sets must be the same as the data type of 'Expression' or at least be convertible to the same data type.
BEGIN END Statements
Using Compound Statements, In some cases, the C/AL syntax only allows you to use a single statement.
If however you have to execute more than one simple statement, the statements can be written as a compound statement by enclosing the statements between the keywords BEGIN and END.
END
The individual statements are separated by a semicolon. In C/AL a semicolon is used to separate statements, and not, as in other programming languages, as a terminator symbol for a statement. Nevertheless, an extra semicolon before an END does not cause an error because it is interpreted by the compiler as an empty statement.
Blocks
The BEGIN-END structure is also called a block. Blocks can be very useful in connection with the other control structures in C/AL
Tuesday, July 21, 2009
C/AL Control Structures
The control structures in C/AL are divided into the following main groups:
1. Compound statements
2. Conditional statements
3. Repetitive statements
4. WITH statements
System-Defined Variables
C/SIDE automatically declares and initializes a number of variables that you can use when you develop applications. The following details describes the system-defined variables.
Rec
When a record is modified, this variable specifies the current record, including the changes that are made.
xRec
When a record is modified, this variable specifies the original values of the record, before the changes.
CurrForm
This variable specifies the current form. You can access the controls of the form through this variable and set the dynamic properties of the form and its controls.
CurrReport
This variable specifies the current report.
RequestOptionsForm
This variable specifies the request options form for the current report.
CurrFieldNo
This variable specifies the field number of the current field in the current form. Retained for compatibility reasons.
Accounts in General Posting Setup
The description and use of Different GL Accounts in General Setup is given below.
Direct Cost Applied Account -
To post direct cost incurred in purchasing materials, capacity and subcontractor's services.
Overhead Applied Account -
To post indirect cost incurred in purchasing materials, capacity and subcontractor's services.
Inventory Account -
To post inventory value of purchased items and manufactured items.
Purchase Variance Account -
To post the difference between standard cost and direct cost of purchased inventory.
Inventory Account (Interim) -
To post expected inventory value. Use together with Inventory Accrual Account (Interim).
WIP Account - To post value of WIP Inventory.
Manufacturing Overhead Variance Account -
To post the difference between actual overhead cost incurred in manufacturing the item and the standard overhead cost for the item.
Capacity Overhead Variance Account -
To post the difference between the overhead cost of capacity consumed and the overhead (standard) cost of capacity setup in the routing for the manufactured item.
COGS Account (Interim) -
To post expected inventory decrease due to sales. Expected sales is posted when post ship without invoice. When Invoice is posted, cost in COGS (Interim) will be reversed out and posted to COGS account.
Inventory Adjustment Account -
To post inventory increase / decrease due to adjustement, revaluation and rounding
Saturday, July 18, 2009
Bulk Inserts
Microsoft Dynamics NAV automatically buffers inserts in order to send them to SQL Server at one time. By using bulk inserts the number of server calls is reduced, thereby improving performance. Bulk inserts also improve scalability by delaying the actual insert until the last possible moment in the transaction. This reduces the amount of time that tables are locked; especially tables that contain SIFT indexes.
Application developers who want to write high performance code that utilizes this feature should understand the following bulk insert constraints.
Bulk Insert Constraints
If you want to write code that uses the bulk insert functionality, you must be aware of the following constraints. Records are sent to SQL Server when the following occurs:
You call COMMIT to commit the transaction.
You call MODIFY or DELETE on the table.
You call any FIND or CALC methods on the table.
Records are not buffered if any of the following conditions are met:
The application is using the return value from an INSERT call; for example,
"IF (GLEntry.INSERT) THEN".
The table that you are going to insert the records into contains any of the following:
BLOB fields
Variant fields
RecordID fields
Fields with the AutoIncrement property set to Yes
The following code example cannot use buffered inserts because it contains a FIND call on the GL/Entry table within the loop.
IF (JnlLine.FIND('-')) THEN BEGIN
GLEntry.LOCKTABLE;
REPEAT IF (GLEntry.FINDLAST) THEN
GLEntry.NEXT := GLEntry."Entry No." + 1ELSEGLEntry.NEXT := 1;// The FIND call will flush the buffered records.GLEntry."Entry No." := GLEntry.NEXT ;
GLEntry.INSERT;
UNTIL (JnlLine.FIND('>') = 0)END;
COMMIT;
If you rewrite the code, as shown in the following example, you can use buffered inserts.
IF (JnlLine.FIND('-')) THEN BEGIN
GLEntry.LOCKTABLE;
IF (GLEntry.FINDLAST) THEN
GLEntry.Next := GLEntry."Entry No." + 1ELSEGLEntry.Next := 1;
REPEAT GLEntry."Entry No.":= GLEntry.Next;
GLEntry.Next := GLEntry."Entry No." + 1;GLEntry.INSERT;
UNTIL (JnlLine.FIND('>') = 0)END;
COMMIT;// The inserts are performed here.
TESTFIELD & VALIDATE Functions
CALCFIELDS, CALCSUMS Functions In Navision
When you use FlowFields in C/AL functions, you must use the CALCFIELDS function to update them.
CALCSUMS calculates the sum of one or more fields that are SumIndexFields in the record.
CALCSUMS has the following syntax.
How to Check the User Name Who Locked the Table?.
Navision allow you to check which user is locking other users.
Step 1 #. Go to File-->Database-->Information.
Step 2 #. Select the Sessions tab.
Step 3#. Drill down to the current sessions.
Step 4#. Check on the Blocking user id column to find out who is locking other people.
Thursday, July 16, 2009
GETRANGEMIN, GETRANGEMAX Functions
GETRANGEMIN retrieves the minimum value of the filter range that is currently applied to a field.
GETRANGEMIN has the following syntax.
Record.GETRANGEMIN(Field);
A runtime error occurs if the filter that is currently applied is not a range. For example, you can set a filter as follows.
Customer.SETFILTER("No.",'100002000030000');
With this filter, the following code fails because the filter is not a range.
BottomValue := Customer.GETRANGEMIN("No.");
GETRANGEMAX Function
GETRANGEMAX has the following syntax.
Value := Record.GETRANGEMAX(Field)
SETCURRENTKEY, SETRANGE, SETFILTER Functions.
#. SETCURRENTKEY
#. SETRANGE
#. SETFILTER
These functions are some of the most commonly used C/AL functions. They set limits on the value of one or more specified fields, so that only a subset of the records are displayed, modified, deleted, and so on.
SETCURRENTKEY Function
SETCURRENTKEY selects a key for a record and sets the sort order that is used for the table in question.
SETCURRENTKEY has the following syntax.
[Ok :=] Record.SETCURRENTKEY(Field1, [Field2],...)
When you use SETCURRENTKEY the following rules apply:
Inactive fields are ignored.
When searching for a key, the first occurrence of the specified field(s) is selected. This means the following:
If you specify only one field as a parameter when you call SETCURRENTKEY, the key that is actually selected may consist of more than one field.
If the field that you specify is the first component of several keys, the key that is selected may not be the key that you expect.
If no keys can be found that include the field(s) that you specify, the return value is FALSE. If you do not test the return value, a runtime error occurs. If you do test the return value, the program will continue to run even though no key was found.
SETRANGE Function
SETRANGE has the following syntax.
Record.SETRANGE(Field [,From-Value] [,To-Value]);
In the following example, SETRANGE filters the Customer table by selecting only those records where the No. field has a value between 10000 and 90000.
Customer.SETRANGE("No.",'10000','90000');
When you use SETRANGE the following rules apply:
SETRANGE removes any filters that were set previously and replaces them with the From-Value and To-Value parameters that you specify.
If you use SETRANGE without setting the From-Value and To-Value parameters, the function removes any filters that are already set.
If you only set the From-Value, the To-Value is set to the same value as the From-Value.
SETFILTER Function
SETFILTER sets a filter in a more general way than SETRANGE.
SETFILTER has the following syntax.
Record.SETFILTER(Field, String [, Value], ...];
Field is the name of the field on which you want to set a filter. String is the filter expression. String may contain placeholders, such as %1 and %2, to indicate where to insert the Value parameter(s) in a filter expression.
The following example selects records where the value of No. is larger than 10000 and not equal to 20000.
Customer.SETFILTER("No.", '>10000 & <> 20000');
If the variables Value1 and Value2 have been assigned "10000" and "20000" respectively, then you can use the following statement to create the same filter.
Customer.SETFILTER("No.",'>%1&<>%2',Value1, Value2);
Wednesday, July 15, 2009
Code Differences in Locking procedure.
A typical use of LOCKTABLE(TRUE,TRUE) in Classic Database Server is shown in the first of the following examples. The equivalent code for the SQL Server Option is shown in the second example. The code that works on both servers is shown in the third example. The RECORDLEVELLOCKING property is used to detect whether record level locking is being used. If this is the case, then you are using the SQL Server Option for Microsoft Dynamics NAV. This is currently the only server that supports record level locking.
Classic Database Server
IF Rec.FIND('-') THEN
UNTIL Rec.NEXT = 0;
Rec.LOCKTABLE(TRUE,TRUE);
IF Rec.FIND('-') THEN
REPEAT
Rec.MODIFY;
UNTIL Rec.NEXT = 0;
SQL Server
Example 2
IF Rec.FIND('-') THEN
REPEAT
UNTIL Rec.NEXT = 0;
IF Rec.FIND('-') THEN
REPEAT
Rec.MODIFY;
UNTIL Rec.NEXT = 0;
Both Server Types
Example 3
IF Rec.RECORDLEVELLOCKING THEN
IF Rec.FIND('-') THEN
REPEAT
UNTIL Rec.NEXT = 0;
IF NOT Rec.RECORDLEVELLOCKING THEN
Rec.LOCKTABLE(TRUE,TRUE);
IF Rec.FIND('-') THEN
REPEAT
Rec.MODIFY;
UNTIL Rec.NEXT = 0;
Locking in Microsoft Dynamics NAV
1. Classic Database Server
2. The SQL Server Option
Both Server Options
Locking
In the beginning of a transaction, the data that you read is not locked. This means that reading data does not conflict with transactions that modify the same data. If you want to ensure that you are reading the latest data from a table, you must lock the table before you read it.
Locking Single Records
Usually, you must not lock a record before you read it even though you may want to modify or delete it afterward. When you try to modify or delete the record, you get an error message if another transaction has modified or deleted the record. You receive this error message because C/SIDE checks the timestamp that it keeps on all the records in a database and detects that the timestamp on the copy you have read is different from the timestamp on the modified record in the database.
Locking Record Sets
Usually, you lock a table before reading a set of records in that table if you want to read these records again to modify or delete them. You must lock the table to ensure that another transaction does not modify these records.
You will not receive an error message if you do not lock the table even though the records have been modified as a result of other transactions being carried out while you were reading them.
Minimizing Deadlocks
To minimize the amount of deadlocks that occur, you must lock records and tables in the same order for all transactions. You can also try to locate areas where you lock more records and tables than you actually need, and then diminish the extent of these locks or remove them completely. This can prevent conflicts from occurring that involve these records and tables.
If this does not prevent deadlocks, you can, lock more records and tables to prevent transactions from running concurrently.
If you cannot prevent the occurrence of deadlocks by programming, you must run the deadlocking transactions separately.
Locking in Microsoft SQL Server
This topic explains how database locking works in Microsoft Dynamics NAV with Microsoft SQL Server.
Locking in SQL Server
When data is read without locking, you get the latest (possibly uncommitted) data from the database. If you call Rec.LOCKTABLE, nothing happens. However, when data is read from the table after LOCKTABLE has been called, the data is locked.
If you call INSERT, MODIFY, or DELETE, the specified record is locked immediately. This means that two transactions, which either insert, modify, or delete separate records in the same table do not conflict. Furthermore, locks are also placed when data is read from the table after the modifying function has been called.
SumIndexFields are maintained when INSERT, MODIFY, or DELETE is called. SQL Server places locks on the records to be updated in the underlying Indexed View. For example, if the application contains a SIFT index on a key consisting of only 'AccountNo' then only one user at the time will be able to modify records on a given AccountNo.
Even though SQL Server initially puts locks on single records, it can also choose to escalate a single record lock to a table lock. This will happen if the overall performance can be improved by not having to set locks on individual records. The improvement in performance must outweigh the loss in concurrency that this excessive locking causes.
If you specify what record to read, for example, by calling Rec.GET, that record is locked. This means that two transactions, which read specific, but separate records in a table does not cause conflicts.
If you browse a record set (read sequentially through a set of records), for example, by calling Rec.FIND('-') or Rec.NEXT, the record set (including the empty space) is locked as you browse through it. This means that two transactions, which just read separate sets of records in a table, will cause a conflict if there are no records between these two record sets. When locks are placed on a record set, other transactions cannot put locks on any record within the set.
Note that C/SIDE determines how many records to retrieve from the server when you ask for the first or the next record within a set. C/SIDE then handles subsequent reads with no additional effort, and fewer calls to the server give better performance. In addition set when you browse.
Microsoft Dynamics NAV with Microsoft SQL Server only supports the default values for the parameters of the LOCKTABLE function – LOCKTABLE(TRUE,FALSE).
Locking in Classic Database Server
Data that is not locked is read from the same snapshot (version) of the database. If you call a modifying function (for example, INSERT, MODIFY, or DELETE), on a table, the whole table is locked.
Locking Record Sets
Usually you lock a table before reading a set of records in that table if you want to read these records again and modify or delete them. With Classic Database Server, you can choose to lock the table with LOCKTABLE(TRUE,TRUE) after reading the records for the first time instead of locking with LOCKTABLE before reading the records for the first time.
When you try to modify or delete the records, you receive an error message if another transaction has modified the records.
You also receive an error message if another transaction has inserted a record into the record set. However, if another transaction has deleted a record from the record set, you will not notice this change. The purpose of locking with LOCKTABLE(TRUE,TRUE) after reading the records for the first time is to improve concurrency by postponing the table lock that Classic Database Server puts on the table.
LOCKTABLE Function
For example, in the beginning of a function, you inspect data in a table and then use this data to perform various checks and calculations. Finally, you want to insert a record based on the result of these calculations. To ensure that your calculations make sense, you must be certain that the values you retrieved at the beginning of the transaction are consistent with the data that is in the table now. You cannot allow other users to update the table while your function is performing its calculations.
LOCKTABLE Function
You can use the LOCKTABLE function to lock the table at the start of your function.
LOCKTABLE has the following syntax.
Record.LOCKTABLE([Wait] [, VersionCheck])
GET, FIND, and NEXT Functions
GET, FIND, and NEXT Functions
The following functions are used to search for records:
. GET
. FIND
. NEXT
These functions are some of the most commonly used C/AL functions. When you search for records, it is important to know the difference between GET and FIND and to know how to use FIND and NEXT in conjunction.
GET Function
GET retrieves one record based on values of the primary key fields.
GET has the following syntax.
[Ok :=] Record.GET([Value],...)
For example, if the No. field is the primary key of the Customer table and if you have created a Record variable called CustomerRec that has a Subtype of Customer, then you can use GET in the following way:
CustomerRec.GET('4711');
The result is that the record of customer 4711 is retrieved.
GET produces a runtime error if it fails and the return value is not checked by the code. In the preceding example, the actual code that you write should be similar to the following.
IF CustomerRec.GET('4711') THEN
.... // Do some processing.
ELSE
.... // Do some error processing.
GET searches for the records, regardless of the current filters, and it does not change any filters. GET always searches through all the records in a table.
FIND Function
FIND locates a record in a C/SIDE table based on the values stored in the keys.
FIND has the following syntax.
Ok := Record.FIND([Which])
The important differences between GET and FIND are the following:
FIND uses the current filters.
FIND can be instructed to look for records where the key value is equal to, greater than, or smaller than the search string.
FIND can find the first or the last record, depending on the sort order defined by the current key.
When you are developing applications in a relational database, there are often one-to-many relationships defined between tables. An example could be the relationship between an Item table, which registers items, and a Sales Line table, which registers the detailed lines from sales orders. One record in the Sales Line table can only be related to one item, but each item can be related to any number of sales line records. You would not want an item record to be deleted as long as there are still open sales orders that include the item. You can use FIND to check for open sales orders.
The OnDelete trigger of the Item table includes the following code that illustrates using FIND.
SalesOrderLine.SETCURRENTKEY(Type,"No.");
SalesOrderLine.SETRANGE(Type,SalesOrderLine.Type::Item);
SalesOrderLine.SETRANGE("No.","No.");
IF SalesOrderLine.FIND('-') THEN
ERROR(Text001,TABLECAPTION,"No.",SalesOrderLine."Document Type");
NEXT Function
NEXT is often used with FIND to step through the records of a table.
NEXT has the following syntax.
Steps := Record.NEXT([Steps])
In the following example, FIND is used to go to the first record of the table. NEXT is used to step through every record, until there are no more. When there are no more records, NEXT returns 0 (zero).
FIND('-');
REPEAT
// process record
UNTIL NEXT = 0;
Essential C/AL Functions
Although there are more than 100 functions in C/AL, there are several functions that you will use more often than the others.
1. GET, FIND, and NEXT Functions
2. SETCURRENTKEY, SETRANGE, SETFILTER, GETRANGEMIN, and GETRANGEMAX Functions
3. INSERT, MODIFY, MODIFYALL, DELETE and DELETEALL Functions
4. LOCKTABLE Function
5. CALCFIELDS, CALCSUMS,FIELDERROR, FIELDNAME, INIT, TESTFIELD, and VALIDATE Functions
6. Progress Windows, MESSAGE, ERROR and CONFIRM Functions
7. STRMENU Function
Guidelines for Placing C/AL Code
In general, place the code as close as possible to the object on which it will operate. This implies that code which modifies records in the database should normally be placed in the triggers of the table fields that are involved.
In reports, always maintain a clear distinction between logical and visual processing and position your C/AL code accordingly. This implies that it is acceptable to have C/AL code in a section trigger if that code controls either the visual appearance of the controls or whether the section should be printed. Never place code that manipulates data in section triggers.
The principle of placing code near the object on which it operates can be overruled in some situations. One reason to overrule this principle is security. Normal users do not have direct access to tables that contain sensitive data, such as the General Ledger Entry table. If you place the code that operates on the general ledger in a codeunit, give the codeunit access to the table, and give the user permission to execute the codeunit, you will not compromise the security of the table and the user will be able to access the table.
There are reasons other than security for putting a posting function in a codeunit. A function that is placed in a codeunit can be called from many places in the application, including, perhaps, some that you did not anticipate when you first designed the application.
Reusing Code
The most important reason for placing C/AL code consistently, and as close to the objects that it manipulates, is that it lets you reuse code. Reusing code makes developing applications both faster and easier. More importantly, if you place your C/AL code as suggested, your applications will be less prone to errors. By centralizing the code, you will not inadvertently create inconsistencies by performing essentially the same calculation in many places
Where to Write C/AL Code
This section describes where to write C/AL code. Almost every object in C/SIDE contains triggers where you can place your C/AL code. Triggers exist for the following objects:
The main Objects in Navision are
1. Tables
2. Table fields
3. Forms, including request forms
4. Form controls
5. Reports
6. Data items
7. Sections
8. Pages
You can initiate the execution of your C/AL code from the following:
#. Command buttons
#. Menu items
#. Codeunits.
Friday, July 10, 2009
Brief About Dynamics NAV 2009
This one is the most important release of Microsoft Dynamics NAV ever, as it brings a completely new architecture, a shiny new user interface, web-services enablement and much more.
The product is currently available in worldwide (W1) version and 13 localized releases in 9 languages.
Unlike any previous versions, this one is not focused on delivering new ERP functionality. From functional perspective it’s the same application as Microsoft Dynamics NAV 5.0 SP1, and no new application features have been introduced.
This has been a strategic move by Microsoft to help customers migrate to new platform easily without too many costs typically incurred by upgrade projects: customers upgrading from version 5.0 SP1 will merely have to upgrade the user interface. It is because data model and code equivalence of versions 5.0 SP1 and 2009, business logic and data model is something that won’t need an upgrade.
User Interface: The RoleTailored interface is a product of several years of Microsoft’s investment into research of user interaction. RoleTailored interface hides the complexity of underlying business logic from users, exposing only those functions pertaining to user’s role. The new user interface is much more intuitive and self-explanatory. Also, users will have one-click access to most-used functionality through Role Centers, organized dashboards which give direct insight into pending to-dos and tasks requiring user’s attention. Accessing one’s daily work doesn’t require browsing through labyrinths of screens and menus—typically, only what’s needed is shown in every screen, and there are vast personalization capabilities to make it all even simpler.
Web Services Enablement: It is now possible to expose any Microsoft Dynamics NAV functionality as a web service without any programming allow Microsoft Dynamics NAV to be seamlessly integrated into workflows extending far beyond system boundaries. Company’s internal processes, line-of-business applications, business-to-business and business-to-customer interactions can now be streamlined with significantly less investment into development and testing.
New Architecture: A completely new, 3-tiered architecture with new Service Tier introduced, a middle component which executes the business logic. This lays the foundation for more robust, scalable and concurrent solutions. New architecture allows for much more freedom in designing network infrastructure, and there will be less scenarios where Terminal Services were the only viable option. Also, higher scalability is now much easier to achieve, which means that Microsoft Dynamics NAV will be able to sustain growth of customer’s business much longer, preserving the investment.
Microsoft Dynamics NAV 2009 is going to be a hot topic of blogs, news sites, articles and analysts’ reports, as it makes the important step towards a single Microsoft Dynamics ERP platform,which sports the RoleTailored user interface, the new standard for all Microsoft Dynamics ERP products.
Thursday, July 9, 2009
Classic Architecture In NAV 2009
Within the Classic architecture, the Classic client connects to either a Microsoft SQL Server database server or to a Classic database server (the proprietary NAV database server). In the RoleTailored architecture, SQL Server is the only database server.
The Classic client is the one Classic component that remains essential for all Microsoft Dynamics NAV 2009 installations, as a tool for development and administration tasks.
Classic Architecture Components
The components that make up the Classic architecture in Microsoft Dynamics NAV 2009 are:
. Classic Client
. Classic Application Server
. Classic Database Server
RoleTailored Architecture Components
Microsoft Dynamics NAV 2009 provides an intuitive and customizable user interface called the RoleTailored client, which developers, partners, administrators, and super users can customize to support the job functions of different work roles in an organization.
For each role within an organization, the Microsoft Certified Partner or developer creates a customizable Role Center that displays key information required for relevant employees and makes their day-to-day tasks easier to complete. Users run the RoleTailored client to find the information and data entry points their jobs require.
For example, partners and developers can customize the sales order processor Role Center to display an interactive list of customers, daily activities, and sales information, and also give users easy access to their Microsoft Office Outlook e-mail, tasks, and calendar, all within the RoleTailored client.
The RoleTailored client is installed when you select either the Client Option or the Developer Environment Option in Microsoft Dynamics NAV Setup.
Microsoft Dynamics NAV Server
The Microsoft Dynamics NAV Server is a .NET-based Windows Service application that works exclusively with SQL Server databases. It uses the Windows Communication Framework as the communication protocol for RoleTailored clients and for Web services. It can execute multiple client requests in parallel and serve other clients by providing Web service access to authenticated clients.
A key difference between the two-tier architecture with the Classic client and three-tier architecture with the RoleTailored client and the Microsoft Dynamics NAV Server is that business logic runs on the Server instead of the client in the three-tier architecture. A simple example is the FILE.CREATE function. In previous versions of Microsoft Dynamics NAV, files are created on the client when code is run. With the RoleTailored architecture, the files are created on the service itself.
Microsoft Dynamics NAV Server provides an additional layer of security between the clients and the database. It leverages the authentication features of the Windows Communications Framework to provide another layer of user authentication and uses impersonation to ensure that business logic is executed in a process that has been instantiated by the user who submitted the request. This means that authorization and logging of user requests is still performed on a per-user basis. This ensures that all Windows authentication and Microsoft Dynamics NAV 2009 roles and permissions that have been granted to the user are correct. It also ensures that business logic–level auditing is still performed.
Microsoft Dynamics NAV Server is installed when you select either the Server Installation Option or the Developer Environment Installation Option in Microsoft Dynamics NAV Setup.
Database Components
The Database Components installation option in Setup installs the software that configures the Microsoft SQL Server database to work with Microsoft Dynamics NAV 2009.
If SQL Server is not present on the computer where you install the database components, Setup installs SQL Server Express 2005, which is an appropriate database option for prototyping and testing, but will likely prove inadequate for all but the smallest production environments.
Database components are installed when you select the Database Components Installation Option in Microsoft Dynamics NAV Setup.
About RoleTailored Architecture
RoleTailored architecture components are new with Microsoft Dynamics NAV 2009. They work together to make the product more flexible, efficient, scalable, and secure, while also improving the user experience.
The Three Tiers of the RoleTailored Architecture
Presentation level (RoleTailored client)
Business logic and communication level (Microsoft Dynamics NAV Server)
Data level (SQL Server database)
The different tiers can be installed on different computers. You can have multiple instances of any of the components (though usually not on the same computer): multiple clients, multiple servers, and multiple database servers.
Here's a simple installation with two SQL Server database computers, each associated with a single Microsoft Dynamics NAV Server computer, each of which in turn supports two Microsoft Dynamics NAV RoleTailored clients:
RoleTailored Architecture Components
RoleTailored Client
Microsoft Dynamics NAV Server
Database Components and SQL Server
What's New in Microsoft Dynamics NAV 2009
This topic contains information about some of the new features and enhancements in Microsoft Dynamics NAV 2009.
1. Three-tier Architecture
With Microsoft Dynamics NAV 2009, the architecture has changed from a two- to three-tier architecture, enabling the new RoleTailored client and Web services.
2. Customizable, Role-Based User Interface
Microsoft Dynamics NAV 2009 provides a more intuitive and customizable user interface (UI) called the RoleTailored client, which you can modify to support the job functions of different work roles in your organization. For each role that you support, you can create a customizable Role Center that displays key information that is required by that role and makes day-to-day tasks easier to complete. Partners, administrators, and super users can customize the UI for specific roles, and individual users can also further personalize the UI to meet their specific needs.
3. Page Objects
Microsoft Dynamics NAV 2009 includes a new object called a page, which is used in the same way as a form. However, unlike forms, you have the flexibility to display pages in the RoleTailored client or to publish a page using a Web service.
You can also transform your existing form objects to page objects.
4. Improved Report Design and Functionality
Microsoft Dynamics NAV 2009 offers an improved reporting experience. You can now create interactive reports to which you can add charts, graphs, tables, and other data presentation elements that were not available in previous versions of Microsoft Dynamics NAV. You can also save your reports in .pdf or .xls file formats.
5. Business Connections with Web Services
Web services are a standard, widely used method for integrating applications and are supported in Microsoft Dynamics NAV 2009. By implementing Web services, you can access Microsoft Dynamics NAV data and business logic from outside the product in a standard, secure way. This enables you to connect Microsoft Dynamics NAV to other systems within an organization.
6. RoleTailored client control add-ins
RoleTailored client control add-ins extend the RoleTailored client with custom functionality. A control add(Available in NAV2009-SP1)-in is a visual element for displaying and modifying data on RoleTailored client pages.
Saturday, July 4, 2009
Navision Functionality That Can Connect Every Corner Of Business.
- Financial Management
GENERAL LEDGER
• Basic General Ledger
• Allocations
• Budgets
• Account Schedules
• Account Schedules Bar Chart
• Consolidation
• Responsibility Centers
• Basic Extensible Business Reporting
Language (XBRL)
• Change Log
• Intercompany Postings
• Reversal of Postings
• Collapsible Chart of Accounts
RECEIVABLES
• Basic Receivables
• Sales Invoicing
• Sales Order Management
• Sales Invoice Discounts
• Alternative Ship-to’s
• Order Promising
• Shipping Agents
• Sales Return Order Management
• Calendars
• Sales Line Discounting
• Sales Line Pricing
• Campaign Pricing
• Sales Tax
• VAT
• Unapply
• Partial Payment
FIXED ASSETS
• Basic Fixed Assets
• Insurance
• Maintenance
• Fixed Assets Allocations
• Reclassification
PAYABLES
• Basic Payables
• Purchase Invoicing
• Purchase Order Management
• Purchase Invoice Discounts
• Requisition Management
• Alternative Order Addresses
• Purchase Return Order Management
• Purchase Line Discounting
• Purchase Line Pricing
• Drop Shipments
• Unapply
• Partial Payment
• Check Writing
• Bank Reconciliation
Analytics
• Report Generator
• Account Schedules
• Analysis by Dimensions
• Business Analytics for Microsoft Navision - Distribution
INVENTORY MANAGEMENT
• Basic Inventory
• Multiple Locations
• Stockkeeping Units
• Alternative Vendors
• Bills of Materials
• Location Transfers
• Item Substitutions
• Item Cross References
• Nonstock Items
• Item tracking
• Item Charges
• Cycle Counting
• Bin
• Put Away
• Warehouse Receipt
• Pick
• Warehouse Shipment
• Standard Cost Worksheet
• Business Notifications
WAREHOUSE MANAGEMENT
• Warehouse Management Systems
• Internal Picks and Put Aways
• Automated Data Capture Systems
(ADCS)
• Bin Set-up
• Returns Management
• Shipment and Delivery
Manufacturing
BASIC MANUFACTURING
• Production Orders
• Production Bills of Materials
AGILE MANUFACTURING
• Version Management
• Agile Manufacturing
SUPPLY PLANNING
• Basic Supply Planning
• Demand Forecasting
CAPACITY PLANNING
• Basic Capacity Planning
• Machine Centers
• Finite Loading
• Manufacturing Costing
Customer Relationship Management
SALES AND MARKETING
• Contact Management
• Contact Classification
• Campaign Management
• Opportunity Management
• Task Management
• Document Management and Interaction
Log
• Contact Search
• E-Mail Logging for Microsoft Exchange
• Microsoft Outlook Client Integration
SERVICE MANAGEMENT
• Service Item Management
• Service Price Management
• Service Order Management
• Service Contract Management
• Planning and Dispatching
• Job Scheduling• Electronic Payments (ACH) - Human Resource Management
• Payroll
• Direct Deposit
• Communications - CUSTOMIZATION TOOLS
• C/SIDE
• Navision Developer’s Toolkit
• Client Monitor
• Code Coverage
• Navision Debugger
• Navision Application Server
• C/ODBC
• C/FRONT - ADDITIONAL APPLICATION-WIDE GRANULES
• Multiple Currencies
• Multiple Document Languages
• Multiple Languages (Each)
• Extended Text
• Reason Codes
• Basic Dimensions
• Advanced Dimensions
• Reports
• Intrastat
• Payroll
• Direct Deposit
• Communications
5 Ways Microsoft Dynamics NAV Helps You Focus on Your Business
- Microsoft Dynamics NAV connects your entire business.
You can use Microsoft Dynamics NAV to replace as much or as little of your existing system as necessary. You choose from application areas for financial management, manufacturing, distribution, customer relationship management, and e-business. - It lets you run your business the way you want.
A local Microsoft Certified Partner tailors the software to meet your exact needs. The partner works closely with you using our proven methodology and tools. Together, you map out your business processes and identify your competitive strengths. - You get quick answers about your business.
Every time a transaction is posted—anywhere within the system—all customer, vendor, account, and item totals are given in real time. You can zero in on details by filtering information (for example, by date, account, or item number) and always rely on the absolute accuracy of the information. - You get more out of customer relationships.
All your business information—customer, product, inventory, sales—is integrated, so you can make better decisions. You know which accounts need your immediate attention, and which can wait. And you can target your sales and marketing campaigns based on specific criteria, such as sales, contact profiles, and previous interactions. - It helps you master change.
Information is transparent, whether you use Microsoft Dynamics NAV to manage the back office, the warehouse, or the shop floor. If a customer calls to change his order in the last minute, for example, you can check the production status, and adjust plans immediately from the sales order.
Microsoft Dynamics NAV
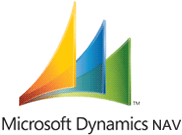
The Official Website For Dynamics- Nav is www.microsoft.com/dynamics/nav/default.mspx